Read this first
Pygame Zero
is a Python library for creating simple video games.
First check that pgzero
is installed on your PC by running this program. Don't worry if you don't understand how it works yet. You will soon learn what all these instructions mean.
# Import the whole pgzrun library.
import pgzrun
# Define the size of the window.
WIDTH = 400
HEIGHT = 300
# Initialize some variables.
text_angle = 0
text_pos = (WIDTH / 2, HEIGHT / 2)
# Callback function to redraw the screen.
def draw():
screen.fill("forestgreen")
screen.draw.text("Welcome to\nPygame Zero!", center=text_pos, fontsize=48, angle=text_angle, color="red", ocolor="white", owidth=2)
# Callback function to update the game. It is called at every frame.
def update():
global text_angle
text_angle -= 0.25
# Callback function to handle mouse clicks.
def on_mouse_down(pos, button):
print("Mouse button", button, "clicked at", pos)
global text_pos
text_pos = pos
# Callback function to handle key presses.
def on_key_down(key, mod):
print("Key down", key, "with mod", mod)
global text_angle, text_pos
if key == keys.SPACE:
text_angle = 0
text_pos = (WIDTH / 2, HEIGHT / 2)
# Start the event loop. This should be the last line of your program.
pgzrun.go()
If all is well, you will see a window like this with the text spinning around:
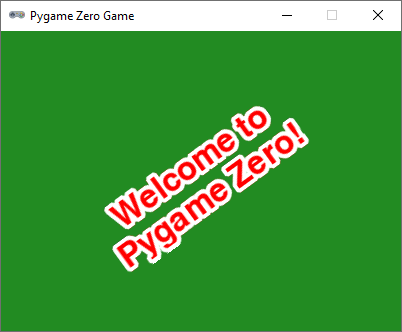
Try clicking the mouse or pressing the space bar and see what happens.
You can also try running this program on repl.it.
Then read these 2 pages to learn the basics.