Read this first
Guizero
is a Python library for creating simple graphical user interfaces (GUIs).
First check that guizero
is installed on your PC by running this program. Don't worry if you don't understand how it works yet. You will soon learn what all these instructions mean.
# Import the widgets we need from the guizero library.
from guizero import App, Text, PushButton, Slider, Combo
# Callback function for when the button is clicked.
def on_click_button():
global click_count
click_count += 1
message.value = f"You clicked {click_count} times"
# Callback function for when the slider is moved.
def on_move_slider(value):
message.text_size = value
# Callback function for when the combo box is changed.
def on_change_combo(value):
message.text_color = value
combo.bg = value
combo.text_color = "white" if value == "blue" else "black"
if __name__ == "__main__":
# Initialize some variables.
click_count = 0
# Create the main window.
app = App(title="Welcome to Guizero", width=400, height=200, bg="#b9f2ff")
# Add some widgets to the main window.
message = Text(app, text="You haven't clicked yet", color="red")
button = PushButton(app, text="Click me!", command=on_click_button)
slider = Slider(app, start=12, end=64, command=on_move_slider)
combo = Combo(app, options=["red", "green", "blue"], command=on_change_combo)
combo.bg = "red"
# Start the event loop. This should be the last line of your program.
app.display()
If all is well, you will see a window like this:
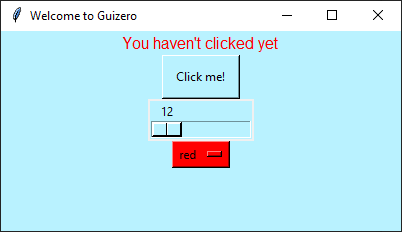
Try clicking the button, moving the slider or choosing a different color and see what happens.
You can also try running this program on repl.it.
Then you should follow the Getting started with GUIs tutorial or the Guizero Workshop tutorial to learn the basics.
The Create Graphical User Interfaces with Python (PDF) book contains a good introduction and has several projects like Wanted Poster, Spy Name Chooser, Meme Generator, Tic-tac-toe, Destroy the Dots, Flood It, Emoji Match, Paint, and more.